C# - Source Generator 소개
이 글의 내용은 Preview 버전을 기준으로 작성돼 현재 유효하지 않습니다. 정식 버전에서의 동작은 다음의 글에 설명했고,
C# -Version 1 Source Generator 실습
; https://www.sysnet.pe.kr/2/0/12985
이 글에 설명한 예제를 Version 1에 맞게 개정한 예제를 "vs2019_sg_sample_v1.zip" 파일로 새롭게 첨부했으니 그 프로젝트를 참고하시면 됩니다. (Visual Studio 2019/2022 모두에서 잘 동작합니다.)
또한, Version 1에 이어 C# 10 컴파일러부터는 Version 2 규약을 새롭게 지원합니다.
C# -IIncrementalGenerator를 적용한 Version 2 Source Generator 실습
; https://www.sysnet.pe.kr/2/0/12986
다음과 같은 소식이 있군요. ^^
Introducing C# Source Generators
; https://devblogs.microsoft.com/dotnet/introducing-c-source-generators/
위의 글에서도 잘 설명하고 있지만, 그대로 따라해 보면서 한글로 다시 소개를 하겠습니다. ^^ 참고로, 저 글을 쓸 당시에는 비주얼 스튜디오에서 지원하지 않았지만, 현재 (16.6.1) 버전에서는 지원하므로 별도의 Preview 버전을 설치하지 않아도 무방합니다. (또한 위의 글에 따르면 .NET 5 Preview도 필요하다는데 제가 테스트한 바로는 .NET Core 3.1.2 환경에서 잘 동작했습니다.)
Source Generator가 뭔지는 위의 글에 포함된 그림에서 잘 설명하고 있습니다.
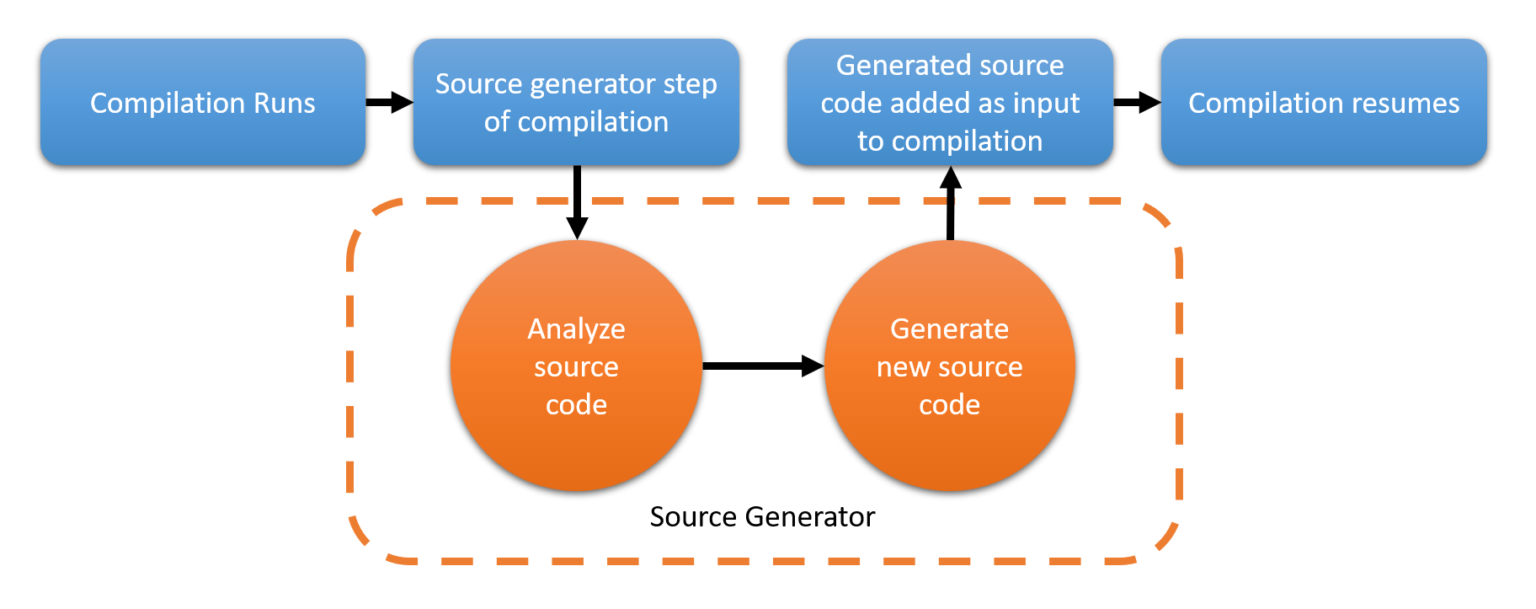
그러니까, 컴파일 시점에 개발자가 임의대로 다시 소스 코드를 구성해 (단, 기존 소스 코드의 변경은 불가능하지만) 끼워 넣을 수 있는 여지를 준 것입니다. 말로만 하면 심심하니 실습을 해보겠습니다.
우선, 콘솔 프로젝트를 하나 만들고 아래의 예제 코드를 입력합니다.
using System;
namespace ConsoleApp1
{
class Program
{
static void Main(string[] args)
{
HelloWorldGenerated.HelloWorld.SayHello();
}
}
}
당연히, 위의 단계까지는 빌드하면 "error CS0103: The name 'HelloWorldGenerated' does not exist in the current context" 오류가 발생합니다. 이제부터 할 일은, 컴파일 시에 HelloWorldGenerated.HelloWorld 타입을 담는 소스 코드를 끼워 넣으면 되는데, 이 작업을 일종의 plug-in처럼 처리하게 됩니다. 이를 위해 ".NET Standard Library" 유형의 프로젝트를 생성하고 다음과 같이 .csproj 파일을 수정합니다.
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Microsoft.CodeAnalysis.CSharp.Workspaces" Version="3.6.0" PrivateAssets="all" />
<PackageReference Include="Microsoft.CodeAnalysis.Analyzers" Version="3.0.0" PrivateAssets="all" />
</ItemGroup>
</Project>
또는 NuGet을 통해 패키지 참조를 해도 됩니다.
Install-Package Microsoft.CodeAnalysis.Analyzers -Version 3.0.0
Install-Package Microsoft.CodeAnalysis.CSharp.Workspaces -Version 3.6.0
이후 ISourceGenerator 인터페이스를 상속받아 "Generator" 특성이 붙은 타입을 정의하면,
using Microsoft.CodeAnalysis;
using Microsoft.CodeAnalysis.Text;
using System;
using System.Text;
namespace HelloGenerator
{
[Generator]
public class MySourceGenerator : ISourceGenerator
{
public void Execute(SourceGeneratorContext context)
{
// begin creating the source we'll inject into the users compilation
var sourceBuilder = new StringBuilder(@"
using System;
namespace HelloWorldGenerated
{
public static class HelloWorld
{
public static void SayHello()
{
Console.WriteLine(""Hello from generated code!"");
Console.WriteLine(""The following syntax trees existed in the compilation that created this program:"");
");
// using the context, get a list of syntax trees in the users compilation
var syntaxTrees = context.Compilation.SyntaxTrees;
// add the filepath of each tree to the class we're building
foreach (SyntaxTree tree in syntaxTrees)
{
sourceBuilder.AppendLine($@"Console.WriteLine(@"" - {tree.FilePath}"");");
}
// finish creating the source to inject
sourceBuilder.Append(@"
}
}
}");
// inject the created source into the users compilation
context.AddSource("helloWorldGenerator", SourceText.From(sourceBuilder.ToString(), Encoding.UTF8));
}
public void Initialize(InitializationContext context)
{
// No initialization required for this one
}
}
}
Roslyn의 컴파일 단계에 참여할 수 있는 plug-in이 완성됩니다. 남은 작업은, 위의 plug-in을 사용할 첫 번째 콘솔 프로젝트에 참조 추가를 하고, Preview 단계의 C# 컴파일러를 사용하도록 지정하면 되는데,
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<OutputType>Exe</OutputType>
<TargetFramework>netcoreapp3.1</TargetFramework>
<LangVersion>preview</LangVersion>
</PropertyGroup>
<ItemGroup>
<ProjectReference Include="..\HelloGenerator\HelloGenerator.csproj" />
</ItemGroup>
</Project>
특별히, plug-in이라는 점을 명시하기 위해 OutputItemType을 Analyzer로 설정하고, 또한 실제 실행 시 필요한 어셈블리는 아니므로 ReferenceOutputAssembly를 false로 지정해 줍니다.
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<OutputType>Exe</OutputType>
<TargetFramework>netcoreapp3.1</TargetFramework>
<LangVersion>preview</LangVersion>
</PropertyGroup>
<ItemGroup>
<ProjectReference Include="..\HelloGenerator\HelloGenerator.csproj" OutputItemType="Analyzer" ReferenceOutputAssembly="false" />
</ItemGroup>
</Project>
이렇게 하고 빌드하면, 컴파일 시점에 ISourceGenerator.Execute 메서드가 호출되고 그로 인해 HelloWorldGenerated.HelloWorld 타입이 정의되어 함께 빌드에 참여하게 되므로 예제 콘솔 프로젝트가 정상적으로 빌드가 됩니다.
그래서 예제 코드를 실행하면 다음과 같은 식의 출력을 볼 수 있습니다.
Hello from generated code!
The following syntax trees existed in the compilation that created this program:
- D:\temp\ConsoleApp1\ConsoleApp1\Program.cs
- D:\temp\ConsoleApp1\ConsoleApp1\obj\Debug\netcoreapp3.1\.NETCoreApp,Version=v3.1.AssemblyAttributes.cs
- D:\temp\ConsoleApp1\ConsoleApp1\obj\Debug\netcoreapp3.1\ConsoleApp1.AssemblyInfo.cs
결과를 보면 알 수 있겠지만, 이러한 출력 결과는 일반적인 C#의 기존 코딩 방법으로는 할 수 없는 작업물입니다.
(첨부 파일은 이 글의 완전한 예제 프로젝트를 포함합니다.)
[이 글에 대해서 여러분들과 의견을 공유하고 싶습니다. 틀리거나 미흡한 부분 또는 의문 사항이 있으시면 언제든 댓글 남겨주십시오.]