C# - VMMap처럼 스택 메모리의 reserve/guard/commit 상태 출력
아래는 VMMap 도구로 .NET 7 콘솔 프로그램의 Main 메서드를 수행한 스레드 스택에 대한 가상 메모리 상태를 보여줍니다.
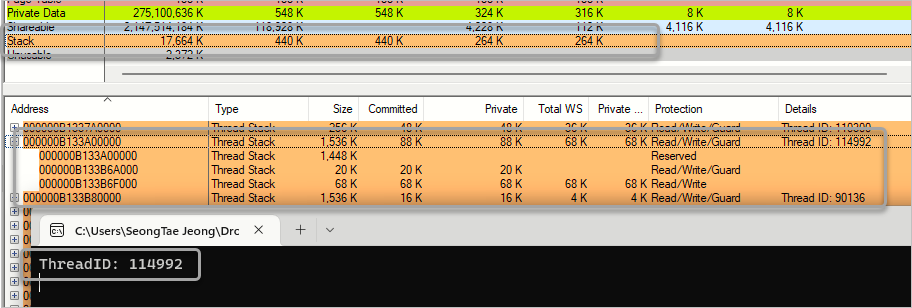
혹시, 저 상황을 우리도 구할 수 있을까요? ^^
이를 위해 우선 구해야 할 값이 (위의 화면에서는 0xB133A00000에 해당하는) 스택의 가상 메모리 시작 주소를 알아내야 합니다. 비록 정확한 시작 주소를 알 수는 없지만, 우리는 스택 주소 범위 내의 주솟값을 ESP/RSP 레지스터를 통해 구할 수 있습니다.
닷넷에서 ESP/RSP 레지스터 값을 구하는 방법
; https://www.sysnet.pe.kr/2/0/13172
하지만, 저렇게 복잡하게 구하지 않아도 (unsafe 예약어를 적용한) 메서드에 포함된 로컬 변수의 값을 이용하는 것으로도 스택 주소 범위 내의 주솟값을 구하는 것이 가능합니다.
static unsafe void Main(string[] args)
{
int dummy = 0;
nuint localVarAdderss = new nuint(&dummy);
Console.WriteLine($"dummy address: 0x{localVarAdderss:x16}");
}
일단 저렇게 스택 범위 내의 주솟값을 구하면, 이를 바탕으로
VirtualQuery Win32 API를 활용해 가상 메모리 할당의 시작 주솟값을 구할 수 있습니다.
using System.Runtime.InteropServices;
internal class Program
{
[DllImport("kernel32.dll", SetLastError = true)]
public static extern int VirtualQuery(UIntPtr lpAddress, ref MEMORY_BASIC_INFORMATION lpBuffer, int dwLength);
static unsafe void Main(string[] args)
{
nuint stackBase = GetThreadStackBasse();
Console.WriteLine($"Stack base: 0x{stackBase:X16}"); // 출력 값: Stack base: 0x00000081E3830000
}
private unsafe static nuint GetThreadStackBasse()
{
MEMORY_BASIC_INFORMATION mbi = new MEMORY_BASIC_INFORMATION();
nuint stackPtr = new nuint((void*)(&mbi));
int result = VirtualQuery(stackPtr, ref mbi, Marshal.SizeOf(mbi));
if (result != 0)
{
return mbi.AllocationBase;
}
return 0;
}
}
[StructLayout(LayoutKind.Sequential)]
public struct MEMORY_BASIC_INFORMATION
{
public UIntPtr BaseAddress;
public UIntPtr AllocationBase;
public uint AllocationProtect;
public IntPtr RegionSize;
public uint State;
public uint Protect;
public uint Type;
}
VirtualQuery가 반환하는 MEMORY_BASIC_INFORMATION 구조체의 AllocationBase가 VMMap에서 보여주는 스택의 시작 주소와 일치합니다.
자, 이렇게 해서 시작 주소를 알았는데요, 이제 스레드 스택의 크기를 알아내야 합니다.
기본 스레드 크기는
PE 헤더의 SizeOfStackReserve로부터 구할 수 있는데, 사용자가 생성한 스레드의 경우에는 그 값을 바꾸는 것이 가능하기 때문에 단순히 SizeOfStackReserve 값을 활용하기에는 범용성이 떨어집니다.
다행히 Windows 8/2012부터는,
GetCurrentThreadStackLimits function (processthreadsapi.h)
; https://learn.microsoft.com/en-us/windows/win32/api/processthreadsapi/nf-processthreadsapi-getcurrentthreadstacklimits
위의 API를 활용해 다음과 같이 구하는 것이 가능합니다.
[DllImport("kernel32.dll")]
static extern void GetCurrentThreadStackLimits(out ulong LowLimit, out ulong HightLimit);
static unsafe void Main(string[] args)
{
GetCurrentThreadStackLimits(out ulong stackLowLimit, out ulong stackHightLimit);
Console.WriteLine($"0x{stackLowLimit:X16} ~ 0x{stackHightLimit:X16}");
}
/* 출력 결과
0x0000003DD5600000 ~ 0x0000003DD5780000
*/
게다가 스택의 시작 주소도 함께 구해주기 때문에 이전에 만들어 둔 GetThreadStackBasse 메서드를 사용할 필요도 없습니다.
자, 이렇게 해서 스택의 시작과 끝을 구했으니 이제 그 사이의 메모리 Page 들이 어떤 속성으로 할당된 것인지 이전에 사용했던 VirtualQuery를 이용해 다음과 같이 구할 수 있습니다.
GetCurrentThreadStackLimits(out ulong stackLowLimit, out ulong stackHightLimit);
for (ulong page = stackLowLimit; page < stackHightLimit;)
{
MEMORY_BASIC_INFORMATION mbi = new MEMORY_BASIC_INFORMATION();
int result = VirtualQuery((nuint)page, ref mbi, Marshal.SizeOf(mbi));
if (result != 0)
{
WritePageInfo((nuint)page, mbi);
}
page += (nuint)mbi.RegionSize;
}
private static void WritePageInfo(nuint page, MEMORY_BASIC_INFORMATION mbi)
{
string text = $"BaseAddress: 0x{mbi.BaseAddress:X16}, Size: {mbi.RegionSize / 1024} KB, {GetPageState(mbi.State)} {GetPageAccess(mbi.Protect)}";
Console.WriteLine($"{text}");
}
private static string GetPageAccess(uint state)
{
StringBuilder sb = new StringBuilder();
if ((state & 0x10) == 0x10)
{
sb.Append("PAGE_EXECUTE, ");
}
if ((state & 0x01) == 0x01)
{
sb.Append("PAGE_NOACCESS, ");
}
if ((state & 0x04) == 0x04)
{
sb.Append("PAGE_READWRITE, ");
}
if ((state & 0x100) == 0x100)
{
sb.Append("PAGE_GUARD");
}
return sb.ToString();
}
private static string GetPageState(uint state)
{
StringBuilder sb = new StringBuilder();
if ((state & 0x1000) == 0x1000)
{
sb.Append("MEM_COMMIT, ");
}
if ((state & 0x10000) == 0x10000)
{
sb.Append("MEM_FREE, ");
}
if ((state & 0x2000) == 0x2000)
{
sb.Append("MEM_RESERVE, ");
}
return sb.ToString();
}
이제 출력 결과를 VMMap과 비교하면,
값이 정확히 일치합니다.
(
첨부 파일은 이 글의 예제 코드를 포함합니다.)
[이 글에 대해서 여러분들과 의견을 공유하고 싶습니다. 틀리거나 미흡한 부분 또는 의문 사항이 있으시면 언제든 댓글 남겨주십시오.]