C# - OpenAI API 사용 - 지원 모델 목록
소소하게 OpenAI에 대해 알아보려고 합니다. ^^
우선, 당연히 API Key를 구해야 하는데요, 이것은 OpenAI 가입 후 "https://platform.openai.com/account/api-keys" 경로에서 생성할 수 있습니다.
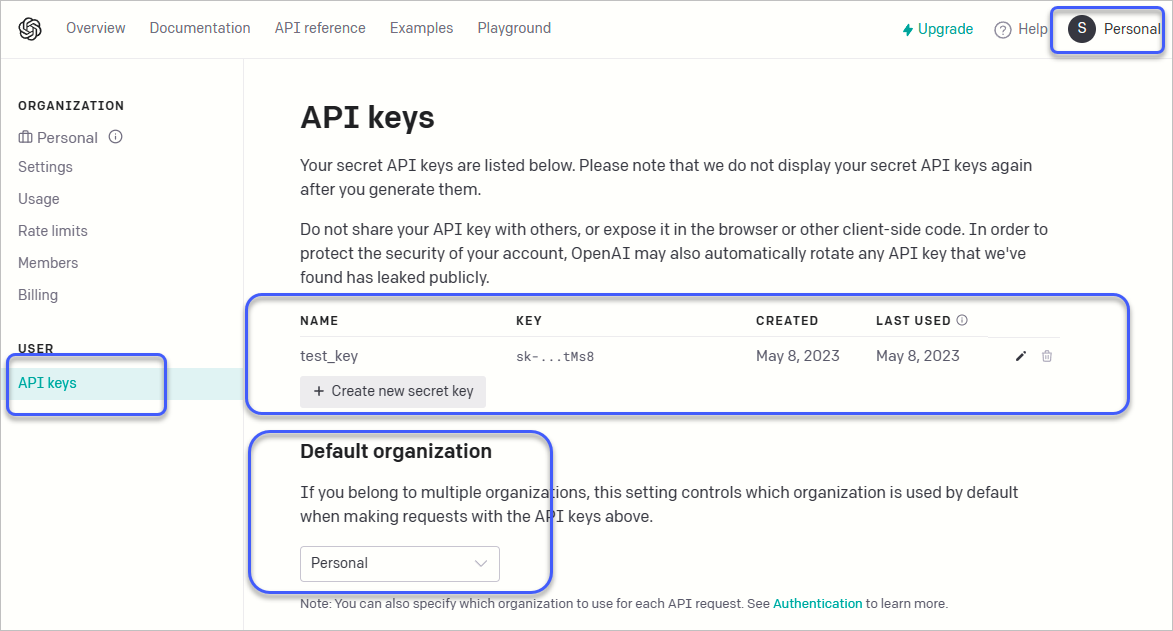
저게 끝입니다. ^^ 이후로는 API Reference 문서를 보며,
API REFERENCE
; https://platform.openai.com/docs/api-reference/introduction
코딩을 하면 되는데요, 이번 글에서는 단순히 OpenAI가 지원하는 Model에 대해서만 구해보겠습니다. 이를 위해 curl을 이용할 수 있으며, 문서에 보면 다음과 같이 전송하면 된다고 나옵니다.
curl https://api.openai.com/v1/models \
-H "Authorization: Bearer $OPENAI_API_KEY" \
-H "OpenAI-Organization: org-UhFLodPvMdmkbjhIFFSgPr7n"
위의 내용에서 OPENAI_API_KEY는 방금 전 생성한 Key이고, OpenAI-Organization은 별도로 "Settings" 메뉴에서 구할 수 있는데요,
"Personal"에 대해서는 동일하게 예제와 같은 "org-UhFLodPvMdmkbjhIFFSgPr7n" 값이기 때문에 그대로 복사해도 됩니다.
curl https://api.openai.com/v1/models -H "Authorization: Bearer ...your_key..." -H "OpenAI-Organization: org-UhFLodPvMdmkbjhIFFSgPr7n"
기본적으로 Personal의 경우 "OpenAI-Organization" 값을 생략해도 API 호출은 잘 동작합니다. 따라서 아래와 같이 수행할 수 있는데요,
C:\temp> curl https://api.openai.com/v1/models -H "Authorization: Bearer sk-YQ...[생략]...Ms8"
{
"object": "list",
"data": [
{
"id": "babbage",
"object": "model",
"created": 1649358449,
"owned_by": "openai",
"permission": [
{
"id": "modelperm-49FUp5v084tBB49tC4z8LPH5",
"object": "model_permission",
"created": 1669085501,
"allow_create_engine": false,
"allow_sampling": true,
"allow_logprobs": true,
"allow_search_indices": false,
"allow_view": true,
"allow_fine_tuning": false,
"organization": "*",
"group": null,
"is_blocking": false
}
],
"root": "babbage",
"parent": null
},
...[생략]...
{
"id": "text-babbage:001",
"object": "model",
"created": 1642018370,
"owned_by": "openai",
"permission": [
{
"id": "snapperm-7oP3WFr9x7qf5xb3eZrVABAH",
"object": "model_permission",
"created": 1642018480,
"allow_create_engine": false,
"allow_sampling": true,
"allow_logprobs": true,
"allow_search_indices": false,
"allow_view": true,
"allow_fine_tuning": false,
"organization": "*",
"group": null,
"is_blocking": false
}
],
"root": "text-babbage:001",
"parent": null
}
]
}
위에서 출력된 값은
https://api.openai.com/v1/models로 질의를 했기 때문에 OpenAI가 지원하는 다양한 AI Model에 해당합니다. C#으로는 기왕이면 ^^ 인텔리센스의 도움을 받을 수 있도록 대략 다음과 같이 코딩하면 좋을 것입니다.
using OpenAI;
using System.Net.Http.Json;
using System.Text.Json.Serialization;
internal class Program
{
static HttpClient _httpClient = new HttpClient();
private static async Task Main(string[] args)
{
string modelUrl = "https://api.openai.com/v1/models";
ModelList? models = await _httpClient.GetFromJsonAsync<ModelList>(modelUrl, SourceGenerationContext.Default.ModelList);
if (models == null)
{
return;
}
foreach (Model model in models.Data)
{
Console.WriteLine($"{model.Id}");
}
}
static Program()
{
(string apiKey, string orgName) = GetKeyInfo(@"d:\settings\openai_key.txt");
_httpClient.DefaultRequestHeaders.Add("Authorization", $"Bearer {apiKey}");
if (string.IsNullOrEmpty(orgName) == false)
{
_httpClient.DefaultRequestHeaders.Add("OpenAI-Organization", orgName);
}
}
private static (string apiKey, string orgName) GetKeyInfo(string filePath)
{
string[] keyInfo = File.ReadLines(filePath).ToArray();
return (keyInfo[0], (keyInfo.Length > 1) ? keyInfo[1] : "");
}
}
namespace OpenAI
{
#if DEBUG
[JsonSourceGenerationOptions(WriteIndented = true)]
#endif
[JsonSerializable(typeof(ModelList))]
[JsonSerializable(typeof(Model))]
[JsonSerializable(typeof(Permission))]
internal partial class SourceGenerationContext : JsonSerializerContext // .NET 6부터 SourceGenerator와 통합된 System.Text.Json
{
}
public class ModelList
{
[JsonPropertyName("object")]
public string Object { get; set; } = "";
[JsonPropertyName("data")]
public List<Model> Data { get; set; } = new List<Model>(); // System.Text.Json의 역직렬화 시 필드/속성 주의
public override string ToString()
{
return $"{Object}: # of data: {Data.Count}";
}
}
public class Model
{
[JsonPropertyName("id")]
public string Id { get; set; } = "";
[JsonPropertyName("object")]
public string Object { get; set; } = "";
[JsonPropertyName("created")]
public int Created { get; set; }
[JsonPropertyName("owned_by")]
public string OwnedBy { get; set; } = "";
[JsonPropertyName("permission")]
public List<Permission> Permissions { get; set; } = new List<Permission>();
[JsonPropertyName("root")]
public string Root { get; set; } = "";
[JsonPropertyName("parent")]
public string Parent { get; set; } = "";
public override string ToString()
{
return $"{Id}, # of permissions: {Permissions.Count}";
}
}
public class Permission
{
[JsonPropertyName("id")]
public string Id { get; set; } = "";
[JsonPropertyName("object")]
public string Object { get; set; } = "";
[JsonPropertyName("created")]
public int Created { get; set; }
[JsonPropertyName("allow_create_engine")]
public bool AllowCreateEngine { get; set; }
[JsonPropertyName("allow_sampling")]
public bool AllowSampling { get; set; }
[JsonPropertyName("allow_logprobs")]
public bool AllowLogprobs { get; set; }
[JsonPropertyName("allow_search_indices")]
public bool AllowSearchIndices { get; set; }
[JsonPropertyName("allow_view")]
public bool AllowView { get; set; }
[JsonPropertyName("allow_fine_tuning")]
public bool AllowFineTuning { get; set; }
[JsonPropertyName("organization")]
public string Organization { get; set; } = "";
[JsonPropertyName("group")]
public string Group { get; set; } = "";
[JsonPropertyName("is_blocking")]
public bool IsBlocking { get; set; }
public override string ToString()
{
return $"{Id}, {Object}";
}
}
}
출력 결과는 2023-05-08 기준으로 다음과 같습니다.
babbage
davinci
text-davinci-edit-001
babbage-code-search-code
text-similarity-babbage-001
code-davinci-edit-001
text-davinci-001
ada
babbage-code-search-text
babbage-similarity
code-search-babbage-text-001
text-curie-001
code-search-babbage-code-001
text-ada-001
text-embedding-ada-002
text-similarity-ada-001
curie-instruct-beta
ada-code-search-code
ada-similarity
code-search-ada-text-001
text-search-ada-query-001
davinci-search-document
ada-code-search-text
text-search-ada-doc-001
davinci-instruct-beta
gpt-3.5-turbo
text-similarity-curie-001
code-search-ada-code-001
ada-search-query
text-search-davinci-query-001
curie-search-query
gpt-3.5-turbo-0301
davinci-search-query
babbage-search-document
ada-search-document
text-search-curie-query-001
whisper-1
text-search-babbage-doc-001
curie-search-document
text-davinci-003
text-search-curie-doc-001
babbage-search-query
text-babbage-001
text-search-davinci-doc-001
text-search-babbage-query-001
curie-similarity
curie
text-similarity-davinci-001
text-davinci-002
davinci-similarity
cushman:2020-05-03
ada:2020-05-03
babbage:2020-05-03
curie:2020-05-03
davinci:2020-05-03
if-davinci-v2
if-curie-v2
if-davinci:3.0.0
davinci-if:3.0.0
davinci-instruct-beta:2.0.0
text-ada:001
text-davinci:001
text-curie:001
text-babbage:001
각각의 특징에 대해서는 다음의 글을 참고하시면 도움이 될 것입니다. ^^
OpenAI API 모델 7가지는 알고 갑시다
; https://iotnbigdata.tistory.com/609
부가적으로, 위의 모델들에 대한 종류를 "Rate limits"에서 확인할 수 있습니다. ^^
재미있는 건, ChatGPT 사이트에서는 "GPT-3.5" 기본값에 "GPT-4"도 사용할 수 있지만 API로는 아직 제공하지 않고 있습니다. 어쨌든 대충 API로 제공되는 Model을 정리하면,
Chat - gpt-3.5-turbo, gpt-3.5-turbo-0301
Moderation - text-moderation-latest, text-moderation-stable
Image - DALL·E 2
Audio - whisper-1
나머지 전부 - Text
이렇게 간단하게 나뉩니다. 오히려 이걸 /v1/models API로 반환한 값으로는 딱히 분류할 기준이 마땅치 않습니다.
(
첨부 파일은 이 글의 예제 코드를 포함합니다.)
참고로, OpenAI API는 무료로
$18 용량이 주어지고, 이후로는 과금을 해야 합니다. 과금은 "Billing" 메뉴에서 신청할 수 있고 월 정액이 아닌, 쓴 만큼 내는 구조인데 최대 설정의 기본값이
$120입니다. (실수로 엄청난 과금 폭탄을 맞지 않을 수 있습니다. ^^)
이에 대해서는 Usage 메뉴에 다음과 같은 표로 쉽게 확인할 수 있습니다.
위의 과금 신청과 ChatGPT의 월 정액
$20 과금은 완전히 별개입니다. (즉, 따로 신청해야 합니다.)
[이 글에 대해서 여러분들과 의견을 공유하고 싶습니다. 틀리거나 미흡한 부분 또는 의문 사항이 있으시면 언제든 댓글 남겨주십시오.]